4716
- 收藏
- 点赞
- 分享
- 举报
ADO2操作数据库代码
[code]// ADO2.cpp: implementation of the CADO class.
//
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "DataBase.h"
#include "ADO2.h"
#include
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
extern CListCtrl m_output_list;
extern int key_flag;
extern _ConnectionPtr m_pConnection;
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
CADO::CADO()
{
}
CADO::~CADO()
{
}
bool CADO::AddBom(CString *aa,int num,CString bb)
{
_variant_t var;
CString str;
_RecordsetPtr myset=NULL;
_RecordsetPtr m_pRecordset;
CString sql;
int i;
m_pRecordset = OpenBom();
sql.Format("SELECT * FROM BomTable where "+bb+"='%s'"\
,aa[0]);
myset=m_pConnection->Execute(_bstr_t(sql),NULL,adCmdText);
if(myset->BOF)
{
m_pRecordset->AddNew();
for(i=0;i
{
m_pRecordset->PutCollect(_variant_t(long(i)),_variant_t(aa));
}
m_pRecordset->Update();
AfxMessageBox("插入成功!");
return TRUE;
}
else
{
return FALSE;
}
}
void CADO::DisplayBom(_RecordsetPtr m_pRecordset,int num)
{
int k=0;
int i;
_variant_t var;
CString str[15];
m_output_list.DeleteAllItems();
while(!m_pRecordset->adoEOF)
{
m_output_list.InsertItem(k,"");
for(i=0;i
{
var = m_pRecordset->GetCollect(_variant_t((long)i));
if(var.vt != VT_NULL)
str = (LPCSTR)_bstr_t(var);
m_output_list.SetItemText(k,i,(LPCSTR)str);
}
k++;
m_pRecordset->MoveNext();
}
}
_RecordsetPtr CADO::OpenBom()
{
m_pRecordsetBom.CreateInstance(__uuidof(Recordset));
// 在ADO操作中建议语句中要常用try...catch()来捕获错误信息,
// 因为它有时会经常出现一些意想不到的错误。jingzhou xu
try
{
m_pRecordsetBom->Open("SELECT * FROM ContractTable", // 查询BomTable表中所有字段
m_pConnection.GetInterfacePtr(), // 获取库接库的IDispatch指针
adOpenDynamic,
adLockOptimistic,
adCmdText);
}
catch(_com_error *e)
{
AfxMessageBox(e->ErrorMessage());
}
return m_pRecordsetBom;
}
_RecordsetPtr CADO::FindBom(int flag,CString *aa,CString *s,int num)
{
_variant_t var;
CString str;
_RecordsetPtr myset=NULL;
_RecordsetPtr m_pRecordset;
CString sql;
int i;
m_pRecordset = OpenBom();
for ( i=1;i
{
if (flag == i)
{
if (aa[i-1] == "")
{
AfxMessageBox(""+s[i-1]+"不能为空!");
return NULL;
}
else
{
sql.Format("SELECT * FROM BomTable where "+s[i-1]+"='%s'"\
,aa[i-1]);
}
break;
}
}
if( i == num+1)
{
AfxMessageBox("请先选择查找项!");
return NULL;
}
myset=m_pConnection->Execute(_bstr_t(sql),NULL,adCmdText);
return myset;
}
bool CADO::modiBom(CString *aa,int num,CString bb)
{
_variant_t var;
CString str;
_RecordsetPtr m_pRecordset;
int i;
m_pRecordset = OpenBom();
m_pRecordset->MoveFirst();
while(1)
{
var= m_pRecordset->GetCollect(_variant_t((long)0));
str=(LPCSTR)_bstr_t(var);
if(strcmp(aa[0],str))
m_pRecordset->MoveNext();
else break;
}
for(i=1;i
{
m_pRecordset->PutCollect(_variant_t((long)i),_variant_t(aa));
}
m_pRecordset->Update();
m_pRecordset->Close();
m_pRecordset = NULL;
return TRUE;
}
bool CADO::DelBom(CString Bom_num)
{
_variant_t var;
CString str;
_RecordsetPtr m_pRecordset;
m_pRecordset = OpenBom();
m_pRecordset->MoveFirst();
while(1)
{
var= m_pRecordset->GetCollect(_variant_t((long)0));
str=(LPCSTR)_bstr_t(var);
if(strcmp(Bom_num,str))
m_pRecordset->MoveNext();
else break;
}
m_pRecordset->Delete(adAffectCurrent); // 参数adAffectCurrent为删除当前记录
m_pRecordset->Update();
m_pRecordset->Close();
m_pRecordset = NULL;
return TRUE;
}
void CADO::LeadInBom(CString *p,int n,CString *s,int num)
{
int count;
int i,j;
int a[20] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0};
int b[20] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0};
CString *q;
_variant_t var;
CString str[20];
_RecordsetPtr myset=NULL;
_RecordsetPtr m_pRecordset;
CString sql;
count = m_output_list.GetItemCount();
if (count<0)
AfxMessageBox("ERROR");
for(i=0;i
{
q=p;
for(j=0;j
{
if(!strcmp(s,*(q++)))
{
a=1;b=j;
break;
}
}
}
for(i=0;i
{
for(j=0;j
{
if(a[j]==1)
{
var=m_output_list.GetItemText(i,b[j]);
if(var.vt != VT_NULL)
str[j] = (LPCSTR)_bstr_t(var);
}
}
if (str[0] != "")
{
m_pRecordset = OpenBom();
sql.Format("SELECT * FROM BomTable where "+s[0]+"='%s'"\
,str[0]);
myset=m_pConnection->Execute(_bstr_t(sql),NULL,adCmdText);
if(myset->BOF)
{
m_pRecordset->AddNew();
for(i=0;i
{
m_pRecordset->PutCollect(_variant_t((long)i),_variant_t(str));
}
m_pRecordset->Update();
}
}
}
}
void CADO::DelAllBom()
{
_RecordsetPtr m_pRecordset;
m_pRecordset = OpenBom();
if(!m_pRecordset->BOF)
{
m_pRecordset->MoveFirst(); // 从0开始
while (m_pRecordset->GetadoEOF()!=VARIANT_TRUE)
{
m_pRecordset->Delete(adAffectCurrent); // 参数adAffectCurrent为删除当前记录
m_pRecordset->Update();
m_pRecordset->MoveFirst(); // 从0开始
}
}
}[/code]
//
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "DataBase.h"
#include "ADO2.h"
#include
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
extern CListCtrl m_output_list;
extern int key_flag;
extern _ConnectionPtr m_pConnection;
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
CADO::CADO()
{
}
CADO::~CADO()
{
}
bool CADO::AddBom(CString *aa,int num,CString bb)
{
_variant_t var;
CString str;
_RecordsetPtr myset=NULL;
_RecordsetPtr m_pRecordset;
CString sql;
int i;
m_pRecordset = OpenBom();
sql.Format("SELECT * FROM BomTable where "+bb+"='%s'"\
,aa[0]);
myset=m_pConnection->Execute(_bstr_t(sql),NULL,adCmdText);
if(myset->BOF)
{
m_pRecordset->AddNew();
for(i=0;i
m_pRecordset->PutCollect(_variant_t(long(i)),_variant_t(aa));
}
m_pRecordset->Update();
AfxMessageBox("插入成功!");
return TRUE;
}
else
{
return FALSE;
}
}
void CADO::DisplayBom(_RecordsetPtr m_pRecordset,int num)
{
int k=0;
int i;
_variant_t var;
CString str[15];
m_output_list.DeleteAllItems();
while(!m_pRecordset->adoEOF)
{
m_output_list.InsertItem(k,"");
for(i=0;i
var = m_pRecordset->GetCollect(_variant_t((long)i));
if(var.vt != VT_NULL)
str = (LPCSTR)_bstr_t(var);
m_output_list.SetItemText(k,i,(LPCSTR)str);
}
k++;
m_pRecordset->MoveNext();
}
}
_RecordsetPtr CADO::OpenBom()
{
m_pRecordsetBom.CreateInstance(__uuidof(Recordset));
// 在ADO操作中建议语句中要常用try...catch()来捕获错误信息,
// 因为它有时会经常出现一些意想不到的错误。jingzhou xu
try
{
m_pRecordsetBom->Open("SELECT * FROM ContractTable", // 查询BomTable表中所有字段
m_pConnection.GetInterfacePtr(), // 获取库接库的IDispatch指针
adOpenDynamic,
adLockOptimistic,
adCmdText);
}
catch(_com_error *e)
{
AfxMessageBox(e->ErrorMessage());
}
return m_pRecordsetBom;
}
_RecordsetPtr CADO::FindBom(int flag,CString *aa,CString *s,int num)
{
_variant_t var;
CString str;
_RecordsetPtr myset=NULL;
_RecordsetPtr m_pRecordset;
CString sql;
int i;
m_pRecordset = OpenBom();
for ( i=1;i
if (flag == i)
{
if (aa[i-1] == "")
{
AfxMessageBox(""+s[i-1]+"不能为空!");
return NULL;
}
else
{
sql.Format("SELECT * FROM BomTable where "+s[i-1]+"='%s'"\
,aa[i-1]);
}
break;
}
}
if( i == num+1)
{
AfxMessageBox("请先选择查找项!");
return NULL;
}
myset=m_pConnection->Execute(_bstr_t(sql),NULL,adCmdText);
return myset;
}
bool CADO::modiBom(CString *aa,int num,CString bb)
{
_variant_t var;
CString str;
_RecordsetPtr m_pRecordset;
int i;
m_pRecordset = OpenBom();
m_pRecordset->MoveFirst();
while(1)
{
var= m_pRecordset->GetCollect(_variant_t((long)0));
str=(LPCSTR)_bstr_t(var);
if(strcmp(aa[0],str))
m_pRecordset->MoveNext();
else break;
}
for(i=1;i
m_pRecordset->PutCollect(_variant_t((long)i),_variant_t(aa));
}
m_pRecordset->Update();
m_pRecordset->Close();
m_pRecordset = NULL;
return TRUE;
}
bool CADO::DelBom(CString Bom_num)
{
_variant_t var;
CString str;
_RecordsetPtr m_pRecordset;
m_pRecordset = OpenBom();
m_pRecordset->MoveFirst();
while(1)
{
var= m_pRecordset->GetCollect(_variant_t((long)0));
str=(LPCSTR)_bstr_t(var);
if(strcmp(Bom_num,str))
m_pRecordset->MoveNext();
else break;
}
m_pRecordset->Delete(adAffectCurrent); // 参数adAffectCurrent为删除当前记录
m_pRecordset->Update();
m_pRecordset->Close();
m_pRecordset = NULL;
return TRUE;
}
void CADO::LeadInBom(CString *p,int n,CString *s,int num)
{
int count;
int i,j;
int a[20] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0};
int b[20] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0};
CString *q;
_variant_t var;
CString str[20];
_RecordsetPtr myset=NULL;
_RecordsetPtr m_pRecordset;
CString sql;
count = m_output_list.GetItemCount();
if (count<0)
AfxMessageBox("ERROR");
for(i=0;i
q=p;
for(j=0;j
if(!strcmp(s,*(q++)))
{
a=1;b=j;
break;
}
}
}
for(i=0;i
for(j=0;j
if(a[j]==1)
{
var=m_output_list.GetItemText(i,b[j]);
if(var.vt != VT_NULL)
str[j] = (LPCSTR)_bstr_t(var);
}
}
if (str[0] != "")
{
m_pRecordset = OpenBom();
sql.Format("SELECT * FROM BomTable where "+s[0]+"='%s'"\
,str[0]);
myset=m_pConnection->Execute(_bstr_t(sql),NULL,adCmdText);
if(myset->BOF)
{
m_pRecordset->AddNew();
for(i=0;i
m_pRecordset->PutCollect(_variant_t((long)i),_variant_t(str));
}
m_pRecordset->Update();
}
}
}
}
void CADO::DelAllBom()
{
_RecordsetPtr m_pRecordset;
m_pRecordset = OpenBom();
if(!m_pRecordset->BOF)
{
m_pRecordset->MoveFirst(); // 从0开始
while (m_pRecordset->GetadoEOF()!=VARIANT_TRUE)
{
m_pRecordset->Delete(adAffectCurrent); // 参数adAffectCurrent为删除当前记录
m_pRecordset->Update();
m_pRecordset->MoveFirst(); // 从0开始
}
}
}[/code]
我来回答
回答0个
时间排序
认可量排序
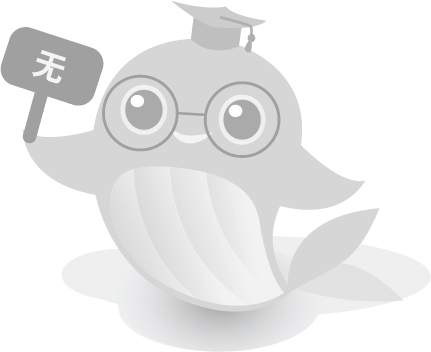
或将文件直接拖到这里
悬赏:
E币
网盘
* 网盘链接:
* 提取码:
悬赏:
E币
Markdown 语法
- 加粗**内容**
- 斜体*内容*
- 删除线~~内容~~
- 引用> 引用内容
- 代码`代码`
- 代码块```编程语言↵代码```
- 链接[链接标题](url)
- 无序列表- 内容
- 有序列表1. 内容
- 缩进内容
- 图片
相关问答
-
2008-12-26 11:30:20
-
2018-12-21 14:40:32
-
2012-12-24 15:27:34
-
2012-12-24 15:28:10
-
2012-12-24 15:24:23
-
2015-08-05 18:54:12
-
2008-05-24 14:52:19
-
2015-08-11 09:47:25
-
2008-08-07 19:00:12
-
2012-12-24 15:29:16
-
2020-11-22 11:33:56
-
02008-07-12 18:46:45
-
2015-08-05 18:05:47
-
2020-11-30 08:40:32
-
2020-12-09 09:23:28
-
2017-07-07 11:01:18
-
2017-06-28 11:37:00
-
2017-11-21 18:46:31
-
2018-12-17 16:40:07
无更多相似问答 去提问

点击登录
-- 积分
-- E币
提问
—
收益
—
被采纳
—
我要提问
切换马甲
上一页
下一页
悬赏问答
-
10海思IVP928
-
522AP10支持F35SQA001G spi nand flash
-
20如何将自有训练的RAW降噪模型应用于AIBNR?
-
10RV1106的低功耗和休眠唤醒功能
-
10海思平台(Hi3516DV500),设置完ldc参数后 工作异常
-
5rv1126-dc-201 安装hi3881.ko wifi驱动成功后 启动connmand服务,系统就崩了
-
10ss928编码的h264帧流,用RTSP传输到电脑,VLC播放不了是什么情况?
-
10我运行rv1126的开发环境后,编译demo提示没有opencv,自行编译安装后没有解决,请问这个怎么处理呢?板子是easy eai nano
-
50CPS-1848 link不上FPGA。
-
10想问一下各位大佬们,关于VI配置成8Lan LVDS模式的相关问题
举报反馈
举报类型
- 内容涉黄/赌/毒
- 内容侵权/抄袭
- 政治相关
- 涉嫌广告
- 侮辱谩骂
- 其他
详细说明
提醒
你的问题还没有最佳答案,是否结题,结题后将扣除20%的悬赏金
取消
确认
提醒
你的问题还没有最佳答案,是否结题,结题后将根据回答情况扣除相应悬赏金(1回答=1E币)
取消
确认