技术专栏
OpenCV DNN C++ 使用 YOLO 模型推理
引言
YOLO(You Only Look Once)是一种流行的目标检测算法,因其速度快和准确度高而被广泛应用。OpenCV 的 DNN(Deep Neural Networks)模块为我们提供了一个简单易用的 API,用于加载和运行预先训练的深度学习模型。本文将详细介绍如何使用 OpenCV 的 DNN 模块来进行 YOLOv5 的目标检测。
准备工作
使用vcpkg直接安装
核心代码解析
结构体和类定义
struct DetectResult
{
int classId;
float score;
cv::Rect box;
};
class YOLOv5Detector
{
public:
void initConfig(std::string onnxpath, int iw, int ih, float threshold);
void detect(cv::Mat& frame, std::vector<DetectResult>& result);
private:
int input_w = 640;
int input_h = 640;
cv::dnn::Net net;
int threshold_score = 0.25;
};
我们定义了一个名为 DetectResult 的结构体,用于存储检测结果,其中包括目标的类别 ID、得分和边界框。
YOLOv5Detector 类提供了两个主要的公共方法:
initConfig:用于初始化网络模型和一些参数。
detect:用于进行目标检测。
初始化配置
void YOLOv5Detector::initConfig(std::string onnxpath, int iw, int ih, float threshold)
{
this->input_w = iw;
this->input_h = ih;
this->threshold_score = threshold;
this->net = cv::dnn::readNetFromONNX(onnxpath);
}
在 initConfig 方法中,我们主要进行了以下操作:
设置输入图像的宽度和高度(input_w 和 input_h)。
设置目标检测的置信度阈值(threshold_score)。
通过 cv:
:readNetFromONNX 方法加载预训练的 ONNX 模型。
目标检测
void YOLOv5Detector::detect(cv::Mat& frame, std::vector<DetectResult>& results)
{
// 图象预处理 - 格式化操作
int w = frame.cols;
int h = frame.rows;
int _max = std::max(h, w);
cv::Mat image = cv::Mat::zeros(cv::Size(_max, _max), CV_8UC3);
cv::Rect roi(0, 0, w, h);
frame.copyTo(image(roi));
float x_factor = image.cols / 640.0f;
float y_factor = image.rows / 640.0f;
cv::Mat blob = cv::dnn::blobFromImage(image, 1 / 255.0, cv::Size(this->input_w, this->input_h), cv::Scalar(0, 0, 0),
true, false);
this->net.setInput(blob);
cv::Mat preds = this->net.forward();
cv::Mat det_output(preds.size[1], preds.size[2], CV_32F, preds.ptr<float>());
float confidence_threshold = 0.5;
std::vector<cv::Rect> boxes;
std::vector<int> classIds;
std::vector<float> confidences;
for (int i = 0; i < det_output.rows; i++)
{
float confidence = det_output.at<float>(i, 4);
if (confidence < 0.45)
{
continue;
}
cv::Mat classes_scores = det_output.row(i).colRange(5, 8);
cv::Point classIdPoint;
double score;
minMaxLoc(classes_scores, 0, &score, 0, &classIdPoint);
// 置信度 0~1之间
if (score > this->threshold_score)
{
float cx = det_output.at<float>(i, 0);
float cy = det_output.at<float>(i, 1);
float ow = det_output.at<float>(i, 2);
float oh = det_output.at<float>(i, 3);
int x = static_cast<int>((cx - 0.5 * ow) * x_factor);
int y = static_cast<int>((cy - 0.5 * oh) * y_factor);
int width = static_cast<int>(ow * x_factor);
int height = static_cast<int>(oh * y_factor);
cv::Rect box;
box.x = x;
box.y = y;
box.width = width;
box.height = height;
boxes.push_back(box);
classIds.push_back(classIdPoint.x);
confidences.push_back(score);
}
}
// NMS
std::vector<int> indexes;
cv::dnn::NMSBoxes(boxes, confidences, 0.25, 0.45, indexes);
for (size_t i = 0; i < indexes.size(); i++)
{
DetectResult dr;
int index = indexes[i];
int idx = classIds[index];
dr.box = boxes[index];
dr.classId = idx;
dr.score = confidences[index];
cv::rectangle(frame, boxes[index], cv::Scalar(0, 0, 255), 2, 8);
cv::rectangle(frame, cv::Point(boxes[index].tl().x, boxes[index].tl().y - 20),
cv::Point(boxes[index].br().x, boxes[index].tl().y), cv::Scalar(0, 255, 255), -1);
results.push_back(dr);
}
std::ostringstream ss;
std::vector<double> layersTimings;
double freq = cv::getTickFrequency() / 1000.0;
double time = net.getPerfProfile(layersTimings) / freq;
ss << "FPS: " << 1000 / time << " ; time : " << time << " ms";
putText(frame, ss.str(), cv::Point(20, 40), cv::FONT_HERSHEY_PLAIN, 2.0, cv::Scalar(255, 0, 0), 2, 8);
}
在 detect 方法中,我们进行了以下几个关键步骤:
对输入图像进行预处理。
使用 cv:
:blobFromImage 函数创建一个 4 维 blob。
通过 setInput 和 forward 方法进行前向传播,得到预测结果。
然后,我们对预测结果进行解析,通过非极大值抑制(NMS)得到最终的目标检测结果。
参考资料
完整代码
#include <fstream>
#include <iostream>
#include <string>
#include <map>
#include <opencv2/opencv.hpp>
struct DetectResult
{
int classId;
float score;
cv::Rect box;
};
class YOLOv5Detector
{
public:
void initConfig(std::string onnxpath, int iw, int ih, float threshold);
void detect(cv::Mat& frame, std::vector<DetectResult>& result);
private:
int input_w = 640;
int input_h = 640;
cv::dnn::Net net;
int threshold_score = 0.25;
};
void YOLOv5Detector::initConfig(std::string onnxpath, int iw, int ih, float threshold)
{
this->input_w = iw;
this->input_h = ih;
this->threshold_score = threshold;
this->net = cv::dnn::readNetFromONNX(onnxpath);
}
void YOLOv5Detector::detect(cv::Mat& frame, std::vector<DetectResult>& results)
{
// 图象预处理 - 格式化操作
int w = frame.cols;
int h = frame.rows;
int _max = std::max(h, w);
cv::Mat image = cv::Mat::zeros(cv::Size(_max, _max), CV_8UC3);
cv::Rect roi(0, 0, w, h);
frame.copyTo(image(roi));
float x_factor = image.cols / 640.0f;
float y_factor = image.rows / 640.0f;
cv::Mat blob = cv::dnn::blobFromImage(image, 1 / 255.0, cv::Size(this->input_w, this->input_h), cv::Scalar(0, 0, 0),
true, false);
this->net.setInput(blob);
cv::Mat preds = this->net.forward();
cv::Mat det_output(preds.size[1], preds.size[2], CV_32F, preds.ptr<float>());
float confidence_threshold = 0.5;
std::vector<cv::Rect> boxes;
std::vector<int> classIds;
std::vector<float> confidences;
for (int i = 0; i < det_output.rows; i++)
{
float confidence = det_output.at<float>(i, 4);
if (confidence < 0.45)
{
continue;
}
cv::Mat classes_scores = det_output.row(i).colRange(5, 8);
cv::Point classIdPoint;
double score;
minMaxLoc(classes_scores, 0, &score, 0, &classIdPoint);
// 置信度 0~1之间
if (score > this->threshold_score)
{
float cx = det_output.at<float>(i, 0);
float cy = det_output.at<float>(i, 1);
float ow = det_output.at<float>(i, 2);
float oh = det_output.at<float>(i, 3);
int x = static_cast<int>((cx - 0.5 * ow) * x_factor);
int y = static_cast<int>((cy - 0.5 * oh) * y_factor);
int width = static_cast<int>(ow * x_factor);
int height = static_cast<int>(oh * y_factor);
cv::Rect box;
box.x = x;
box.y = y;
box.width = width;
box.height = height;
boxes.push_back(box);
classIds.push_back(classIdPoint.x);
confidences.push_back(score);
}
}
// NMS
std::vector<int> indexes;
cv::dnn::NMSBoxes(boxes, confidences, 0.25, 0.45, indexes);
for (size_t i = 0; i < indexes.size(); i++)
{
DetectResult dr;
int index = indexes[i];
int idx = classIds[index];
dr.box = boxes[index];
dr.classId = idx;
dr.score = confidences[index];
cv::rectangle(frame, boxes[index], cv::Scalar(0, 0, 255), 2, 8);
cv::rectangle(frame, cv::Point(boxes[index].tl().x, boxes[index].tl().y - 20),
cv::Point(boxes[index].br().x, boxes[index].tl().y), cv::Scalar(0, 255, 255), -1);
results.push_back(dr);
}
std::ostringstream ss;
std::vector<double> layersTimings;
double freq = cv::getTickFrequency() / 1000.0;
double time = net.getPerfProfile(layersTimings) / freq;
ss << "FPS: " << 1000 / time << " ; time : " << time << " ms";
putText(frame, ss.str(), cv::Point(20, 40), cv::FONT_HERSHEY_PLAIN, 2.0, cv::Scalar(255, 0, 0), 2, 8);
}
std::map<int, std::string> classNames = {{0, "-1"}, {1, "0"}, {2, "1"}};
int main(int argc, char* argv[])
{
std::shared_ptr<YOLOv5Detector> detector = std::make_shared<YOLOv5Detector>();
detector->initConfig(R"(D:\AllCodeProjects\best.onnx)", 640, 640, 0.25f);
cv::Mat frame = cv::imread(R"(D:\0002.jpg)");
std::vector<DetectResult> results;
detector->detect(frame, results);
for (DetectResult& dr : results)
{
cv::Rect box = dr.box;
cv::putText(frame, classNames[dr.classId], cv::Point(box.tl().x, box.tl().y - 10), cv::FONT_HERSHEY_SIMPLEX,
.5, cv::Scalar(0, 0, 0));
}
cv::imshow("OpenCV DNN", frame);
cv::waitKey();
results.clear()
声明:本文内容由易百纳平台入驻作者撰写,文章观点仅代表作者本人,不代表易百纳立场。如有内容侵权或者其他问题,请联系本站进行删除。
红包
点赞
收藏
评论
打赏
- 分享
- 举报
评论
0个
手气红包
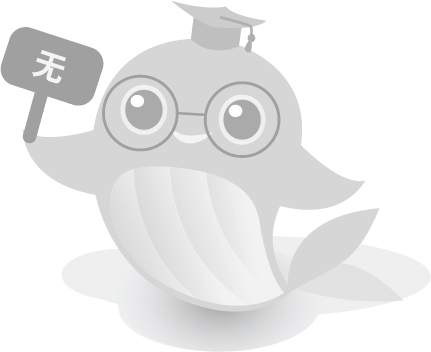
相关专栏
-
浏览量:343次2023-12-19 16:06:28
-
浏览量:8162次2020-12-16 13:01:00
-
浏览量:320次2023-06-03 16:03:04
-
浏览量:5845次2021-06-07 11:48:50
-
浏览量:127次2024-01-22 15:27:25
-
浏览量:199次2024-02-28 15:36:09
-
浏览量:959次2023-04-12 19:12:22
-
浏览量:853次2023-04-12 18:59:36
-
浏览量:7187次2021-01-13 17:06:49
-
浏览量:287次2023-11-13 11:08:23
-
2023-04-14 10:20:01
-
浏览量:359次2024-02-02 18:15:06
-
浏览量:204次2023-12-14 16:51:13
-
浏览量:150次2024-03-05 15:05:36
-
浏览量:94次2024-01-24 16:33:36
-
浏览量:207次2024-02-04 10:08:58
-
浏览量:114次2024-01-22 17:02:06
-
浏览量:4516次2021-03-15 16:24:28
-
浏览量:142次2024-02-19 17:07:05
置顶时间设置
结束时间
删除原因
-
广告/SPAM
-
恶意灌水
-
违规内容
-
文不对题
-
重复发帖
打赏作者
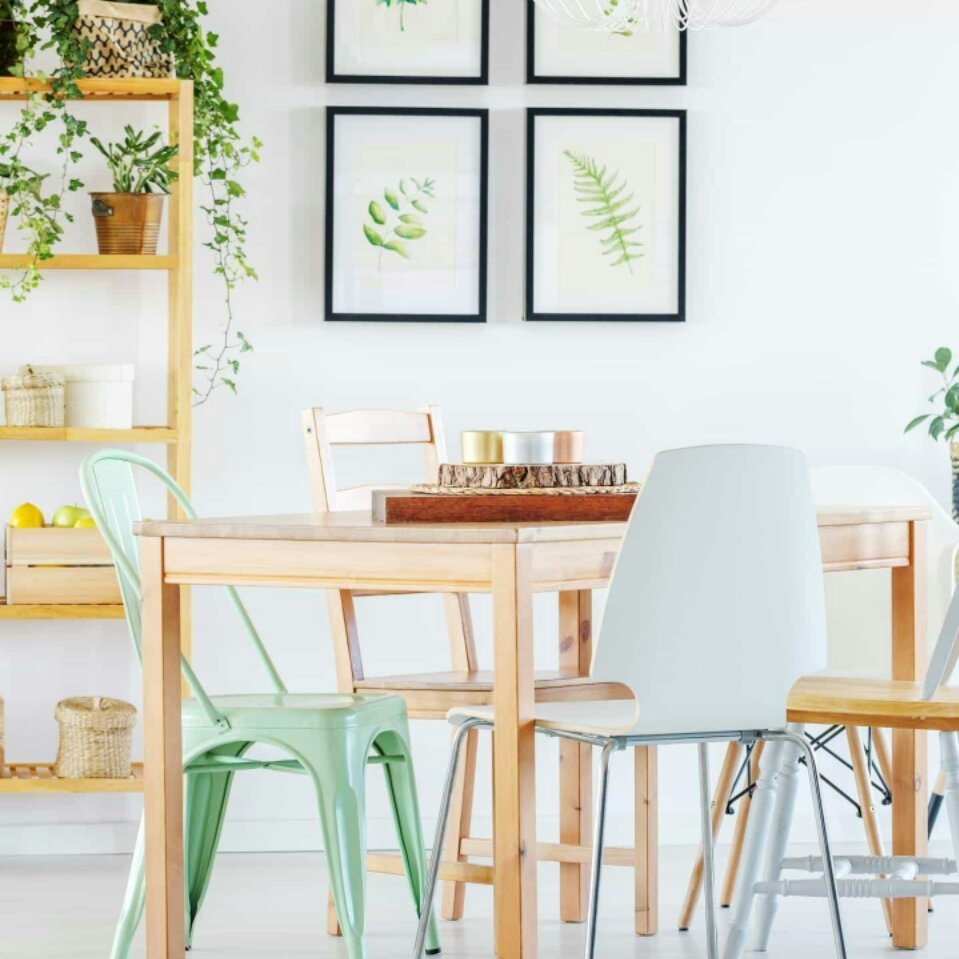
郭金**
您的支持将鼓励我继续创作!
打赏金额:
¥1

¥5

¥10

¥50

¥100

支付方式:
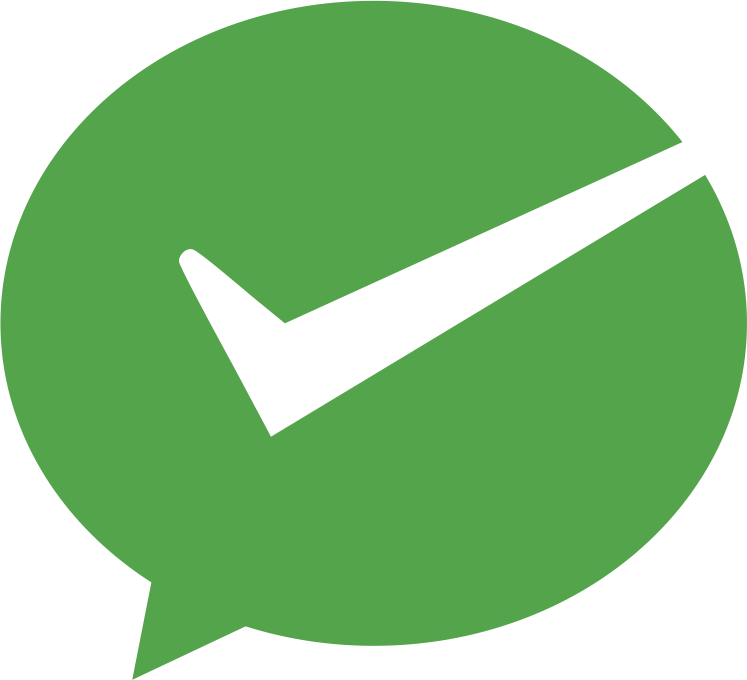
举报反馈
举报类型
- 内容涉黄/赌/毒
- 内容侵权/抄袭
- 政治相关
- 涉嫌广告
- 侮辱谩骂
- 其他
详细说明
审核成功
发布时间设置
发布时间:
请选择发布时间设置
是否关联周任务-专栏模块
审核失败
失败原因
请选择失败原因
备注
请输入备注